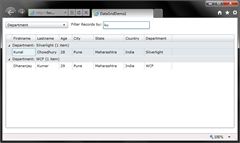
In my last article, we discussed about “Grouping Records in Silverlight DataGrid using PagedCollectionView” where we grouped our data records by column name using the PagedCollectionView.
In this article, we will see how this PagedCollectionView will help us to filter out the records based on the search keyword. Not much code but the trick done with the same class will do everything for us in some easy way. We will learn about this here in this article. Not only this, we will also see the filtering on top of the grouped records too. Read the complete article to see it in action.
Background
If you didn’t read my previous article, I will ask you to read that article (“Grouping Records in Silverlight DataGrid using PagedCollectionView”) first and then come to read this one. It will help you in many cases to understand the basic requirement and the code.
Now come to the actual article. In some cases we need to filter the DataGrid records based on the search key. A DataGrid may contain a huge collection of data and in that case, finding a particular record is very difficult. In such time, you need to filter the records.
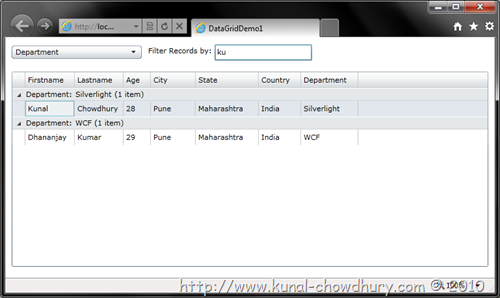
In this article, we will implement the same filtering feature in some quick easy steps.
XAML Design
First of all, we need to redesign our existing XAML page with a Search TextBox as shown below:
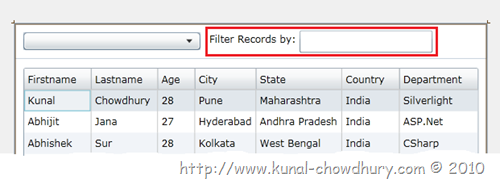
To do this, we need a TextBlock (which is optional) to label the TextBox. The TextBox is a mandatory field where you will be able to insert the search query. Whenever the end user types something here, the DataGrid will filter the records.
Here is the complete XAML for your reference:
<UserControl
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:viewModels="clr-namespace:DataGridDemo1.ViewModels"
xmlns:sdk="http://schemas.microsoft.com/winfx/2006/xaml/presentation/sdk"
x:Class="DataGridDemo1.Views.MainView">
<UserControl.Resources>
<viewModels:MainViewModel x:Key="MainViewModel"/>
</UserControl.Resources>
<StackPanel x:Name="LayoutRoot" Background="White"
DataContext="{StaticResource MainViewModel}">
<StackPanel Orientation="Horizontal">
<ComboBox Width="200" Height="20" Margin="10"
HorizontalAlignment="Left"
SelectionChanged="ComboBox_SelectionChanged">
<ComboBoxItem Content="City"/>
<ComboBoxItem Content="State"/>
<ComboBoxItem Content="Department"/>
</ComboBox>
<TextBlock Text="Filter Records by: " Height="20"/>
<TextBox Width="150" Height="25" TextChanged="TextBox_TextChanged"/>
</StackPanel>
<sdk:DataGrid
IsReadOnly="True" Margin="10" Height="300" ItemsSource="{Binding Employees}"/>
</StackPanel>
</UserControl>
You will see that, not a big change there. We used one extra StackPanel to make our application UI layout clean and proper.
Playing with the Code
It’s time for us to write the code inside the ViewModel to do the filtering. Make sure that, we will use the class named “PagedCollectionView” which we used earlier. Hence, we are familiar with the collection too.
Add a private string variable called “searchKey” which we will use internally for our feature implementation. Now, write a new method “FilterDataBySearchKey()” passing the search key to it to store in the member variable.
You will notice that, the PagedCollectionView has a property called “Filter” which takes Predicate. This Filter predicate will do the tricks for our requirement. Here, our PagedCollectionView is our collection named “Employees”. First of all set the Filter of this collection to null to remove the previous filtering. Then set a new Predicate function call to it. Inside the predicate action, do your filtering mechanism.
Have a look into the below ViewModel code to understand the same:
private string searchKey;
/// <summary>
/// Filters the data by search key.
/// </summary>
/// <param name="searchKey">The search key.</param>
public void FilterDataBySearchKey(string searchKey)
{
this.searchKey = searchKey;
Employees.Filter = null;
Employees.Filter = new Predicate<object>(FilterRecords);
}
/// <summary>
/// Filters the records.
/// </summary>
/// <param name="obj">The obj.</param>
/// <returns></returns>
private bool FilterRecords(object obj)
{
var employee = obj as Employee;
return employee != null &&
(employee.Firstname.ToLower().IndexOf(searchKey) >= 0 ||
employee.Lastname.ToLower().IndexOf(searchKey) >= 0 ||
employee.City.ToLower().IndexOf(searchKey) >= 0 ||
employee.State.ToLower().IndexOf(searchKey) >= 0);
}
You will see that the FilterRecords() is the actual predicate to the PagedCollectionsView’s Filter key. Here we searched whether the “searchKey” is present in the Firstname, Lastname, City or State by using the IndexOf(). This will search anywhere in the value for the matching key.
If you want to implement it for the first characters of string, you can use StartsWith() too. We are searching for only Firstname, Lastname, City and State. You can include more or reduce some too from the actual logic.
As our actual code is ready, we need to integrate that with the TextChanged event. To do this, go to the code behind file and inside the TextBox_TextChanged event, get the search Key from the TextBox’s Text and pass it to the viewmodel’s method.
Here is the same code:
private void TextBox_TextChanged(object sender, TextChangedEventArgs e)
{
string searchKey = (sender as TextBox).Text.Trim().ToLower();
(Resources["MainViewModel"] as MainViewModel).FilterDataBySearchKey(searchKey);
}
Up to this, our full code has been implemented. No more code require for our sample. Lets start running the application to see it in action, so that, we will be able to understand whether our code is working properly or not.
Playing with the Application
Lets build the source and run the application. You will see the same UI as we demoed in the previous article. Only change here is a TextBox which asks to enter the search key to filter the records.
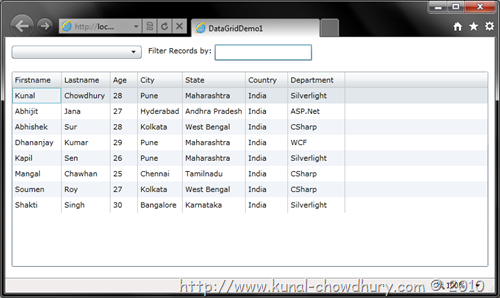
Start typing something in the Filter box. You will notice that, the records are automatically filtering out as per the entered term. This filtering is happening for both Firstname, Lastname, City and State as we implemented the logic for that only.
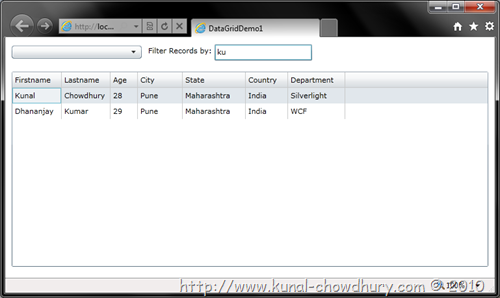
Nice, right? Wondering whether it will work for the grouped data? Lets have a try. Group the records by department and then type something in the search box.
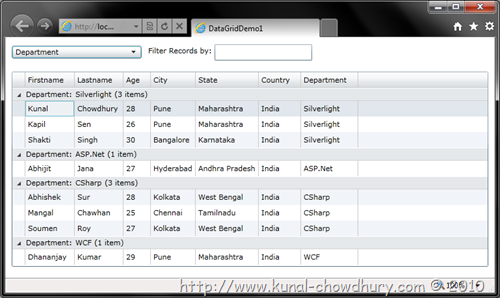
You will see that, the records are now filtered based on the key but in grouped manner i.e. department wise.
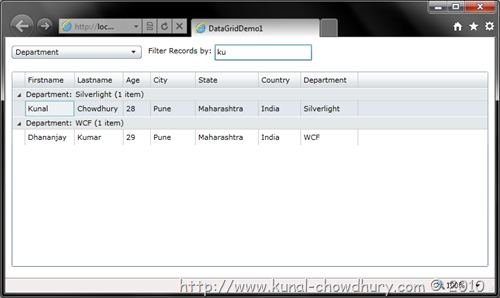
Modify to a different search term. You will notice the change in a fraction of second as and when you type something there in the search box.
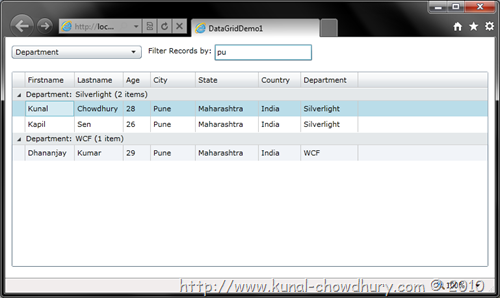
We noticed that, all the filtering is working with the value starting with the searched key. Let us check how it works for the searched key which is in the middle of the value.
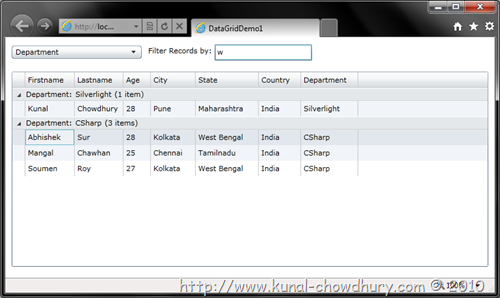
Enter ‘w’ in the TextBox and you will see that the records which has ‘w’ inside any value, has been filtered properly.
Download
Demo
End Note
Hope, this article was also interesting & helpful for you like the previous one. Wish this will help you in future to filter out the DataGrid records. Many more things are there with DataGrid. I will post another article on the same soon and that will also help you another importance of the same PagedCollectionView. Explore more to do lots of magic with DataGrid.
Don’t forget to provide your feedback/suggestion. If you have any issue, drop a line here. I will try to answer you as early as possible. Cheers.