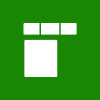
If you are working with the Telerik’s RadRichTextBox control for Silverlight, you might come to a situation where as per your business need you might have to customize the default context menu of the said control. If you are looking for an way to add or remove context menu group, this post will help you.
Continue reading the post to understand the way this context menu has been structured. Once you understand the basics, it will be easy for you to do further modifications.
Introduction
On a sunny afternoon when I was gossiping with few of my friends in the cafeteria, one of my friend mentioned that, today he has a complex task creating a custom context menu for one of the Telerik’s Silverlight control named “RadRichTextBox”.
Before going further, let me tell you that, RadRichTextBox control is part of the Telerik’s Silverlight Rad library and comes inside “Telerik.Windows.Controls.RichTextBoxUI” assembly. To use this control, you need three more assembly references in your project named “Telerik.Windows.Controls”, “Telerik.Windows.Controls.Navigation” and “Telerik.Windows.Documents” as shown below:
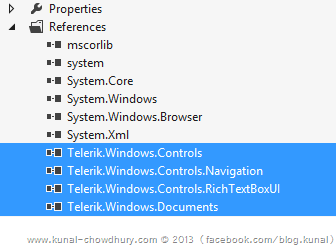
So the discussion started with several question and answer sessions to understand the current implementation of the same. It was not so easy that I thought initially. The discussion was as followed:
Me: Do you have the handle of the context menu?
A: The context menu is internal to the RadRichTextBox control and we don’t have any direct handle to it.
Me: Do you want to recreate the context menu from scratch?
A: Might be, if we don’t find the actual handle of the same and that will be very difficult.
Me: Why is it so difficult?
A: It needs lot of works to take care the control’s internal implementation.
Me: Can you structure out the context menu of the control?
A: Definitely! The context menu of the RadRichTextBox control has various groups that shows or hides based on various parameters. If you right click on a blank area or on top of a word (spelled correctly), you will see a basic context menu having three groups. The first group has Cut, Copy and Paste menu items; the second group has a Hyperlink menu item to insert hyperlink in the document and the third group has two menu items named Font and Paragraph for document formatting.
When you right click on a wrongly spelled word, you will see two different groups for spell check and dictionary in the same context menu. Similarly when you right click on a table, you will see another group of menu items for table specific operations in the same context menu.
Here is a screenshot of different view of the same context menu of RadRichTextBox control:
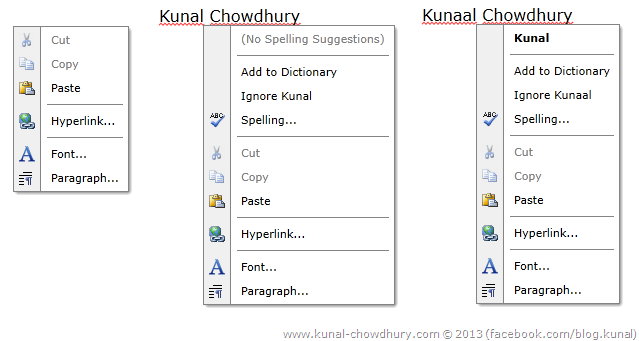
Me: Ah, ok! Then there might be some way to handle this. There should be some groups that Telerik uses to show or hide the menu groups.
Finally after doing a bit of analysis, we came to a solution where the analysis worked for the requirement and thus thought to share the same with you so that you can find it easy enough. If you came to this page after searching online tutorials, this will help you to understand the logic. Just follow the same steps to add or remove menu items dynamically (as per your business need) in the RadRichTextBox context menu.
Implementation of Code
Telerik exposes an enum named “ContextMenuGroupType” with 12 enum values and is present under the namespace called “Telerik.Windows.Controls.RichTextBoxUI.Menus”. It specifies the type of the context menu items group. The enum declaration is as below:
The context menu exposes an event called “Showing” and takes an object sender and a ContextMenuEventArgs that publicly holds the collection of the context menu groups. Before proceeding to next steps, you must have to register the “Showing” event which we will need next to implement the dynamic add and remove feature of the context menu group.
In the “OnContextMenuShowing” event handler (as shown below), you have to structure out the implmentation for adding and/or removing the context menu group and context menu item:
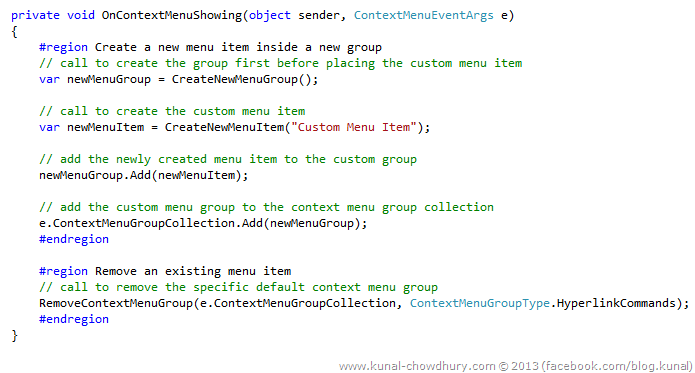
As for the demo, we will first create a new menu group. Then we will create a new menu item with display text as “Custom Menu Item” and will add it to the recently created menu group. As per your need you can create and add as many items that you want in the group.
Next, you have to add the recently created group to the context menu group collection available under the second parameter named “ContextMenuEventArgs”. Add all the groups that you want to display in the context menu. That’s all about adding groups and menu items in the context menu. Don’t worry about adding a separator, the group itself will add the separators in proper place.
Likewise if you want to remove some default groups, you can loop through the context menu group collection and find out the specific group type that you want to remove. In this demo, we searched for HyperlinkCommands group type and removed it from the context menu on demand.
Here is a snapshot of the context menu after adding a custom menu item in a custom menu group (Image 1) and another context menu after deleting the hyperlink group (Image 2):
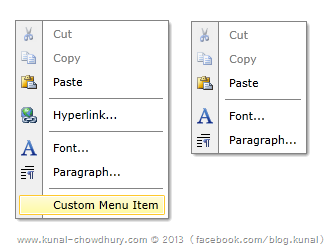
Note that, it deletes the separators of the hyperlink group too with out writing any extra code. For your reference, sharing the complete source code here which will help you to understand the code better:
I hope this will help you to understand the logic behind the context menu of Telerik’s RadRichTextBox control for Silverlight. This might be the same case for WPF too, which I didn’t try. Let me know, if you have any further queries on that and I will try to answer you as soon as I can.
Subscribe to my blog’s RSS feed and email newsletter to get updates about the new post. I am available on Twitter, Facebook and Google+. If you are there, connect me to have all technical discussions and technical updates. Last but not least, happy coding. Cheers!!!