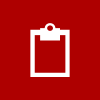
The “Clipboard” is a user driven set of functions that enables an application to transfer data between applications or within an application. Windows 8 Store SDK defines a set of APIs to handle the OS clipboard in your application.
In this continued series of Windows 8 Store application development tutorial, we will learn more about the API and the way to integrate the Clipboard in your WinRT application.

Clipboard API
“Clipboard” is a static class present in the Windows.ApplicationModel.DataTransfer namespace provides users ability to get and set information from the clipboard object. You need to create a DataPackage object while saving data to the clipboard.
Let’s see the meta data of the Clipboard class that we are going to explore today:
public static class Clipboard
{
public static DataPackageView GetContent();
public static void SetContent(DataPackage content);
public static void Flush();
public static void Clear();
public static event EventHandler<object> ContentChanged;
}
The static class Clipboard contains GetContent() method which allows you to get the existing content of the clipboard in your application. The SetContent() method sets the current content that is stored in the clipboard object.
There are four types of data that a user can handle in a clipboard and those are as below:
- Plain Text
- HTML Content
- Images
- Files
Now we will see how to save each of those data into the clipboard from your Windows 8 Store applications.
Saving Plain Text in Clipboard
You will find this example useful in maximum scenarios in your Windows 8 Store application. You will need to copy plain text into clipboard to use the copied content in another part of your application, in a different application or in other desktop applications.
First you need to create an object of DataPackage and set the plain text in it using the SetText() method. Once you populate the data package object with the plain text, call the Clipboard.SetContent() method with the data package as parameter to save the copied text in clipboard as shown below:
public void CopyTextToClipboard(string textToCopy)
{
var dataPackage = new DataPackage();
dataPackage.SetText(textToCopy);
Clipboard.SetContent(dataPackage);
}
Thus, the plain text will be copied and saved in your system clipboard which you can use inside the same application, in a different Windows 8 Store application or in a desktop application.
Saving HTML Content in Clipboard
Sometime you may need to copy a HTML content in your clipboard. This is similar to copying plain text but there are few tricks that you need to perform before setting the content in the clipboard. Here is the code snippet of the same:
public void CopyHtmlContentToClipboard(string htmlTextToCopy)
{
var dataPackage = new DataPackage();
var htmlContent = HtmlFormatHelper.CreateHtmlFormat(htmlTextToCopy);
dataPackage.SetHtmlFormat(htmlContent);
Clipboard.SetContent(dataPackage);
}
First you need to process the html string using the HtmlFormatHelper.CreateHtmlFormat() method and then you have to set the processed string as HTML content in the data package object. Finally, call the SetContent() method of the clipboard API to set the same.
Saving Image in Clipboard
If you need to copy image, you first have to get the image file from the system. You need to call either the method named StorageFile.GetFileFromApplicationUriAsync() if you are loading the image from application folder itself; or call the method named StorageFile.GetFileFromPathAsync(), if you are loading the image from a specific path. Then set the data package by calling the SetBitmap() method as shown in the below code snippet:
public async void CopyImageToClipboard(Uri imageUri)
{
var dataPackage = new DataPackage();
var storageFile = await StorageFile.GetFileFromApplicationUriAsync(imageUri);
dataPackage.SetBitmap(RandomAccessStreamReference.CreateFromFile(storageFile));
Clipboard.SetContent(dataPackage);
}
Once, you are ready with the DataPackage object, call the SetContent() method of the Clipboard class to save the image in the clipboard for later use.
Saving File in Clipboard
If you want to copy a file in a clipboard, you will need to get the file asynchronously either from the application folder or from a different path as mentioned above. Once the storage file is available with you, you need to add it in a list of storage files as shown below:
public async void CopyFileToClipboard(Uri fileUri)
{
var dataPackage = new DataPackage();
var files = new List<StorageFile>();
var storageFile = await StorageFile.GetFileFromApplicationUriAsync(fileUri);
files.Add(storageFile);
dataPackage.SetStorageItems(files);
Clipboard.SetContent(dataPackage);
}
The DataPackage object will have the instance of the storage file list by calling the SetStorageItems() method and finally you have to set the data package object as the content of the clipboard.
Get Clipboard Content
Let’s see how to get the content of the clipboard that you stored in your application or outside your application. Also in this section we will learn how to check the data format type inside the clipboard. You have to call the GetContent() method from the static Clipboard class, which will return you the object of DataPackage as shown below:
var dataPackage = Clipboard.GetContent();
Then you need to check the type of the content that you just got from the Clipboard. This operation you will do in the instance of data package by following the below code snippet:
if (dataPackage.Contains(StandardDataFormats.Text))
{
// handle the text content
}
if (dataPackage.Contains(StandardDataFormats.Html))
{
// handle the HTML content
}
if (dataPackage.Contains(StandardDataFormats.Bitmap))
{
// handle the image content
}
if (dataPackage.Contains(StandardDataFormats.StorageItems))
{
// handle the file content
}
That’s all about fetching content from your system clipboard. In case you need to clear the content present in the clipboard, you can call the Clipboard.Clear() method.
End Note
I hope that, this post will help you to understand the use of clipboard APIs and implement the functionality in your application very easily. Let me know in case any questions on the same.
I am available on Twitter, Facebook and Google+. Connect with me for technology updates and discussions. Don’t forget to subscribe to my blog’s RSS feed and email newsletter if you didn’t do it yet. This will help you to be updated on the latest posts immediately with a notification in your inbox.