Earlier in the TypeScript Tutorial series (Getting started with TypeScript) we learnt about the TypeScript configuration file, variable declaration and basic data types. I hope that was neat and clear to understand the very basics of TypeScript.
Today in this article, we will learn how to define a class and instantiate class object. Continue reading to learn about it today.
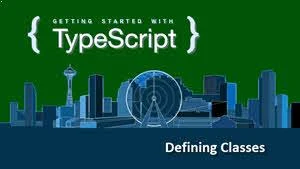
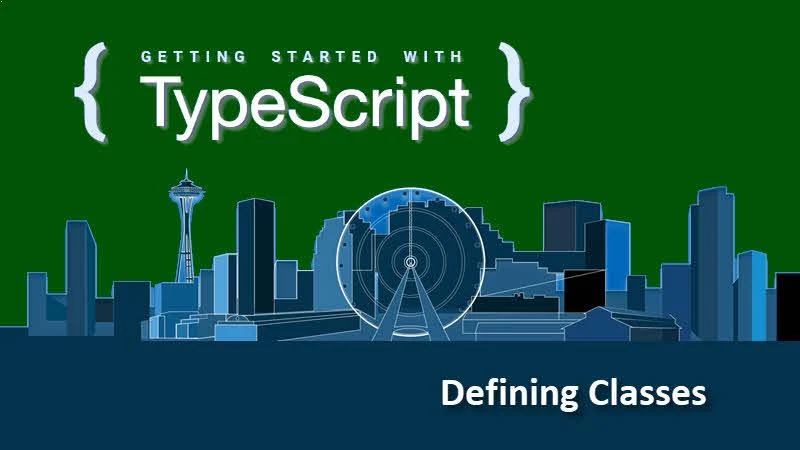
π TypeScript Tutorial - Getting started with TypeScript
Defining a class in TypeScript
In TypeScript, you can use OOPs concept and create classes. Just like C# and other OOPs oriented programming languages, you can define a class with the keyword class
. Let's take the following example into consideration:
class Person {
// properties
firstName: string;
lastName: string;
// default construtor
constructor () {
}
// parameterized construtor
constructor (fName: string, lName: string) {
this.firstName = fName;
this.lastName = lName;
}
// method
getFullName() {
return `${firstName} ${lastName}`;
}
}
In the above example, the class Person
has four members: two properties (firstName
, lastName
), two class constructors (constructor
) and one method (getFullName()
). To access the members of the class, you can use this
operator. For example, this.firstName
, this.getFullName()
etc.
By default all the members in a TypeScript class are public. This is as good as marking the members as public using the public
access modifier. The above class can be written as below, by explicitly marking the members as public
.
class Person {
// properties
public firstName: string;
public lastName: string;
// default construtor
constructor () {
}
// construtor
public constructor (fName: string, lName: string) {
this.firstName = fName;
this.lastName = lName;
}
// method
public getFullName() {
return `${firstName} ${lastName}`;
}
}
Defining constructors in a TypeScript class
A class can have two types of constructors: default constructor and parameterized constructor. In the above example, the first constructor is a default constructor, which takes no/zero parameters. The second one is a parameterized constructor, which takes one or more parameter values (in our case, it takes two inputs):
// default construtor
constructor () {
}
// construtor
public constructor (fName: string, lName: string) {
this.firstName = fName;
this.lastName = lName;
}
In the above example, we have first defined the properties, then passed the values using parameterized constructor and then filled those properties. TypeScript supports automatic property creation. Instead of following all these steps, if you define the constructor parameters as public, TypeScript will do rest of the job for you.
Consider the following example which shows how to define a property using parameterized constructor:
class Person {
// parameterized construtor
constructor (public fName: string, public lName: string) {
}
// method
getFullName() {
return `${firstName} ${lastName}`;
}
}
Instantiating an instance of a TypeScript class
To create the instance of the class and access it's members, you can use new
operator, the way you create a class instance in C# and/or Java. Here's two different approaches to create the class instance:
let person: Person = new Person("Kunal", "Chowdhury");
console.log(person.getFullName());
// alternative way
let person = new Person("Kunal", "Chowdhury");
console.log(person.getFullName());
π TypeScript Tutorial - Getting started with TypeScript
CodeProject